What is Nested Array in Documents?
In MongoDB, documents can contain arrays as values, which can, in turn, contain other arrays or documents.
- In this document, the field skills is an array, containing several string elements.
- The field contacts is a nested array, containing several array elements.
[
{
_id: ObjectId('66053f848ccb6407b4ba21c6'),
name: 'Michael Johnson',
age: 35,
working_experience: 8,
department: 'Finance',
salary: 70000,
skills: [ 'Accounting', 'Financial Analysis', 'Excel' ],
contacts: [
{ type: 'address', value: '123 Street' },
{ type: 'Email', value: 'abc@xyz.com' }
]
}
]
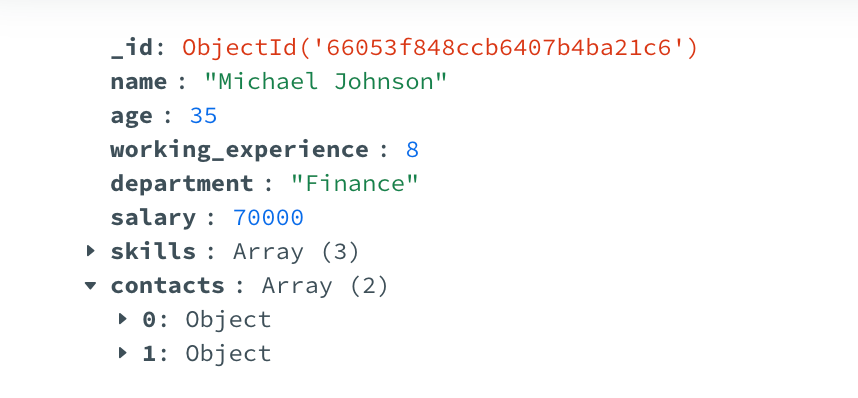
Query Nest Array
You can query documents based on fields within nested arrays using dot notation.
db.personnel.find({"contacts.type": "Email"})
Update Nest Arrays
Updating Elements: Use the positional $set
operator and $
to update specific elements within a nested array.
For example, I want to change the address 123 Street to 789 Street.
> db.personnel.update({"_id":ObjectId("66053f848ccb6407b4ba21c6"),
"contacts.type":"address" },
{ $set: { "contacts.$.value": "789 Street" } } )
#let's check the result
> db.personnel.find({"name":"Michael Johnson"})
[
{
_id: ObjectId('66053f848ccb6407b4ba21c6'),
name: 'Michael Johnson',
age: 35,
working_experience: 8,
department: 'Finance',
salary: 70000,
skills: [ 'Accounting', 'Financial Analysis', 'Excel' ],
contacts: [
{ type: 'address', value: '789 Street' },
{ type: 'Email', value: 'abc@xyz.com' }
]
}
]
Adding Elements: You can use $push
to add elements to a nested array.
For example, I want to add a new address called 456 Street to the employee Michael Johnson.
> db.personnel.update({ "_id": ObjectId("66053f848ccb6407b4ba21c6")},
{ $push: { "contacts":
{ "type": "address", "value": "456 Street" } } } )
#let's check the result
> db.personnel.find({"name":"Michael Johnson"})
[
{
_id: ObjectId('66053f848ccb6407b4ba21c6'),
name: 'Michael Johnson',
age: 35,
working_experience: 8,
department: 'Finance',
salary: 70000,
skills: [ 'Accounting', 'Financial Analysis', 'Excel' ],
contacts: [
{ type: 'address', value: '789 Street' },
{ type: 'Email', value: 'abc@xyz.com' },
{ type: 'address', value: '456 Street' }
]
}
]
Removing Elements: You can use $pull
to remove elements matching a specific condition from a nested array.
For example, I want to remove all the address info from this document.
> db.personnel.update({ "_id": ObjectId('66053f848ccb6407b4ba21c6')},
{$pull:{"contacts":{"type":"address"}}})
# let's check the result
> db.personnel.find({"name":"Michael Johnson"})
[
{
_id: ObjectId('66053f848ccb6407b4ba21c6'),
name: 'Michael Johnson',
age: 35,
working_experience: 8,
department: 'Finance',
salary: 70000,
skills: [ 'Accounting', 'Financial Analysis', 'Excel' ],
contacts: [ { type: 'Email', value: 'abc@xyz.com' } ]
}
]