Objectives:
- Get familiar with variable and module concepts
- Get familiar with Cloud Spanner Product
Prerequisite
- Google Cloud Account
Structure
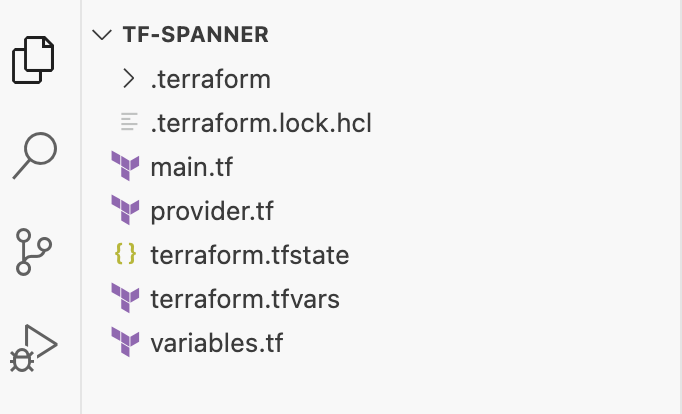
Code
providers.tf
terraform { required_providers { google = { source = "hashicorp/google" version = "~> 4.0" } } } provider "google" { project = var.project_id region = var.region }
main.tf
resource "google_spanner_instance" "db-instance" { name = "terraform-spanner-instance" config = "regional-${var.region}" display_name = "TF Spanner Instance" processing_units = var.processing_units force_destroy = var.force_destroy } resource "google_spanner_database" "test-database" { instance = google_spanner_instance.db-instance.name name = "pets-db" # Can't run destroy unless set to false deletion_protection = var.deletion_protection ddl = [ "CREATE TABLE Owners (OwnerID STRING(36) NOT NULL, OwnerName STRING(MAX) NOT NULL) PRIMARY KEY (OwnerID)", "CREATE TABLE Pets (PetID STRING(36) NOT NULL, OwnerID STRING(36) NOT NULL, PetType STRING(MAX) NOT NULL, PetName STRING(MAX) NOT NULL, Breed STRING(MAX) NOT NULL) PRIMARY KEY (PetID)", ] }
variables.tf
variable "deletion_protection" { description = "If set to true, you cannot run terraform destroy if there are databases created." type = bool default = false } variable "force_destroy" { description = "If set to true, running terraform destroy will delete all backups." type = bool default = true } variable "processing_units" { type = number default = 100 } variable "project_id" { description = "The GCP Project ID." type = string } variable "region" { type = string }
terraform.tfvars
project_id = "Your_Project_ID" region = "Your_Region"
Check the result
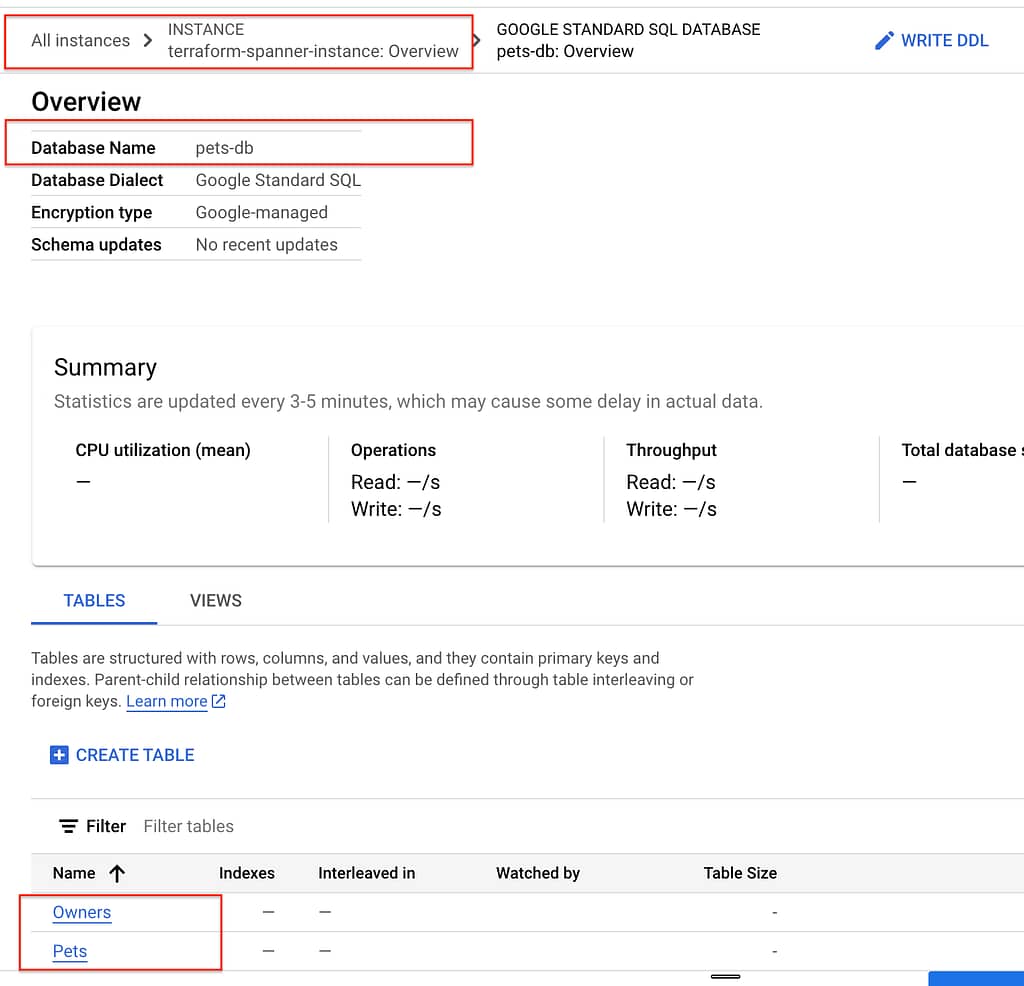