Tasks
- Create a database called company
- Create a collection called personnel
- Insert 10 employee’s info in the collection.
Code
# create a database
USE company
# creata a collection
db.createCollection("personnel")
# define 10 employee's data
var personnelData = [
{
name: "John Doe",
age: 30,
working_experience: 5,
department: "Engineering",
salary: 60000,
skills: ["JavaScript", "Node.js", "MongoDB", "React"]
},
{
name: "Jane Smith",
age: 28,
working_experience: 4,
department: "Marketing",
salary: 55000,
skills: ["Digital Marketing", "SEO", "Social Media Management"]
},
{
name: "Michael Johnson",
age: 35,
working_experience: 8,
department: "Finance",
salary: 70000,
skills: ["Accounting", "Financial Analysis", "Excel"]
},
{
name: "Emily Brown",
age: 32,
working_experience: 6,
department: "Human Resources",
salary: 62000,
skills: ["Recruitment", "Employee Relations", "Training"]
},
{
name: "David Wilson",
age: 40,
working_experience: 12,
department: "Operations",
salary: 75000,
skills: ["Project Management", "Supply Chain", "Lean Six Sigma"]
},
{
name: "Sarah Lee",
age: 27,
working_experience: 3,
department: "Customer Support",
salary: 50000,
skills: ["Customer Service", "Communication", "Troubleshooting"]
},
{
name: "Alex Garcia",
age: 34,
working_experience: 7,
department: "Sales",
salary: 67000,
skills: ["Sales Strategy", "Negotiation", "CRM"]
},
{
name: "Michelle Martinez",
age: 31,
working_experience: 5,
department: "Research and Development",
salary: 63000,
skills: ["Research", "Data Analysis", "Python", "Machine Learning"]
},
{
name: "Daniel Thompson",
age: 29,
working_experience: 4,
department: "Product Management",
salary: 58000,
skills: ["Product Development", "Product Launch", "Market Analysis"]
},
{
name: "Amanda Hall",
age: 33,
working_experience: 9,
department: "Quality Assurance",
salary: 69000,
skills: ["Testing", "Bug Tracking", "Automation"]
}
];
#Insert the sample documents into the 'personnel' collection
db.personnel.insertMany(personnelData);
You can verify it in the Compass. It should look like this:
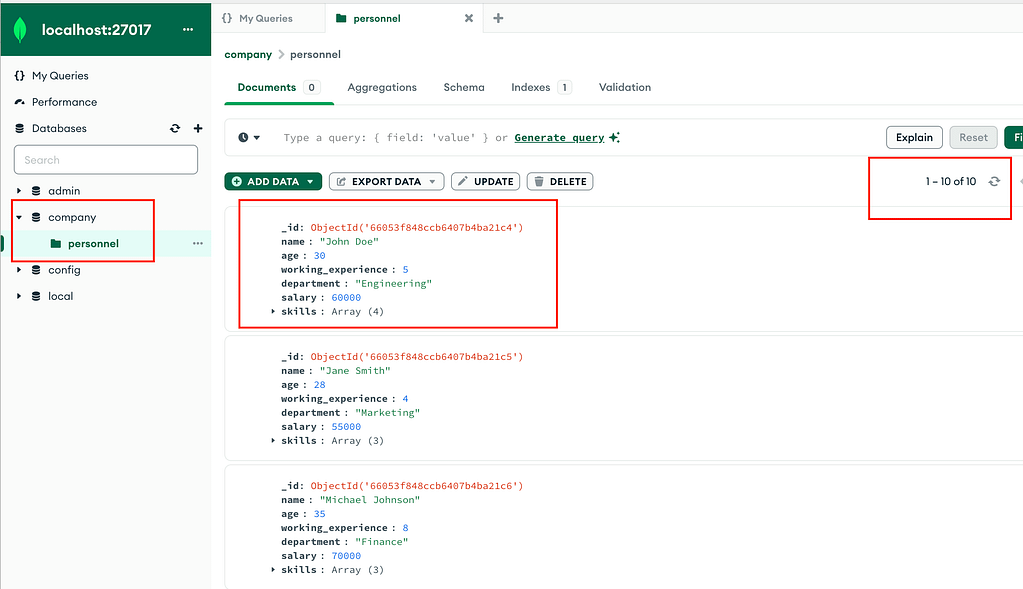