1. Create a Collection
- Create a collection
- Display all collections in the current database
- Access collections property
#Create a new collection called aribnb
> db.createCollection("aribnb")
< { ok: 1 }
# Display all collections in the currently selected database
> show collections
< aribnb
#Access collections property
> db.getCollectionNames()
< ['aribnb']
2. Create and Insert Document
To create a document in MongoDB, you first need to insert it into a collection. For example, I will add one document into the airbnb collection.
You can use insertOne()
to insert one document or use insertMany()
to insert multiple documents
# create a collection called rooms and insert one document into it
> db.rooms.insertOne({
"listing_url": "https://www.airbnb.com/rooms/10006546",
"name": "Ribeira Charming Duplex",
"summary": "Fantastic duplex apartment with three bedrooms, located in the historic area of Porto, Ribeira (Cube)...",
"interaction": "Cot - 10 € / night Dog - € 7,5 / night",
"house_rules": "Make the house your home...",
"property_type": "House",
"room_type": "Entire home/apt",
"bed_type": "Real Bed",
"minimum_nights": "2",
"maximum_nights": "30"})
< {
acknowledged: true,
insertedId: ObjectId('6604b009c49e849d79b781d1')
}
If we check the GUI in MongoDB Compass, it will look like this.
Notice: we did not create or assign the _id
, MongoDB automatically creates one for you. The _id
It is unique and identifies each document in the collection.
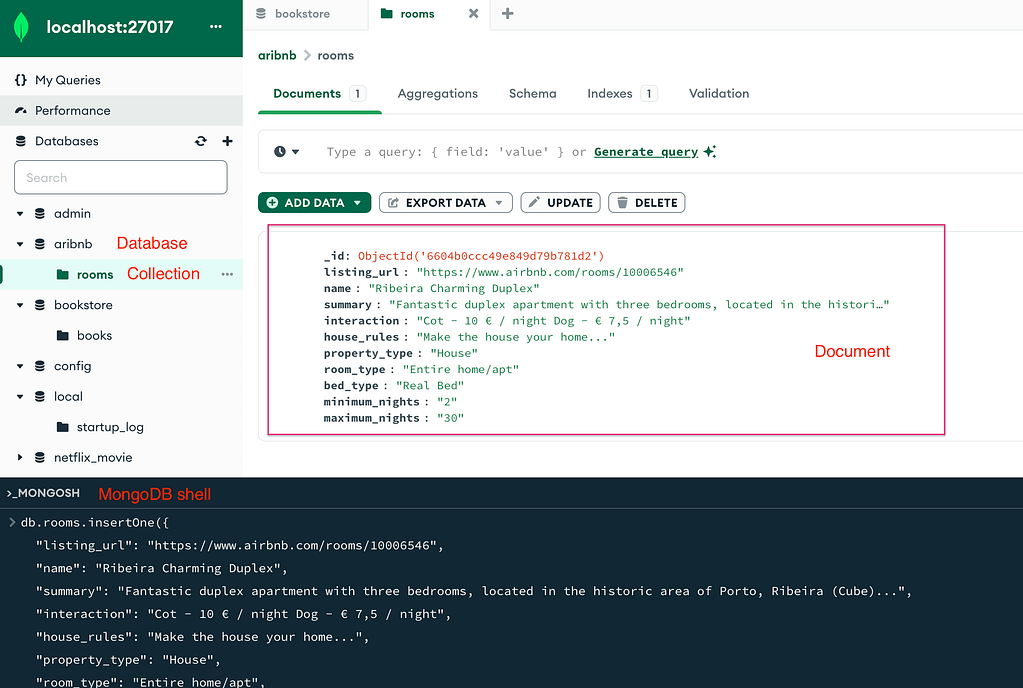
Example of insertMany()
# add three documents into a collection called listings.
db.listings.insertMany([
{
title: "Cozy Apartment in Downtown",
description: "A comfortable apartment located in the heart of the city.",
price: 100,
location: "Downtown",
rating: 4.5
},
{
title: "Luxurious Villa with Ocean View",
description: "Experience luxury with breathtaking ocean views.",
price: 500,
location: "Beachfront",
rating: 5
},
{
title: "Charming Cottage in the Countryside",
description: "Escape the hustle and bustle in this serene countryside cottage.",
price: 200,
location: "Rural",
rating: 4
}
])
# we can see three documents have been added.
< {
acknowledged: true,
insertedIds: {
'0': ObjectId('6604b3d4c49e849d79b781d3'),
'1': ObjectId('6604b3d4c49e849d79b781d4'),
'2': ObjectId('6604b3d4c49e849d79b781d5')
}
}